We assume that you have a multi module project and the screenshot gallery should be aggregated from several modules and published with GitHub pages. You find a working example of the configuration described here in the Screenshot Maven Plugin project it self.
1. Configuration in the parent pom
Define as much as possible in the parent pom
to avoid duplicated configuration.
1.1. Specify the following properties
<properties>
<screenshot-maven-plugin.version>1.0.3-SNAPSHOT</screenshot-maven-plugin.version>
<rootDirectory>${project.basedir}</rootDirectory> (1)
<localRepositoryPath>${rootDirectory}/maven-repo</localRepositoryPath>
<screenshotGalleryTarget>${rootDirectory}/gh-pages/target/gallery-src</screenshotGalleryTarget> (2)
</properties>
1 | I haven’t found any Maven property that specifies the root of the project so I’ve made one up. The rootDirectory
property is redefined in every module with a relative path to the root. Append as many /.. necessary to reach the root.
|
2 | Specify a common directory for gallery screenshots from different modules |
1.2. Add a plugin element to the pluginManagement part
<pluginManagement>
<plugins>
<plugin>
<groupId>se.bluebrim.maven.plugin</groupId>
<artifactId>screenshot-maven-plugin</artifactId>
<version>${screenshot-maven-plugin-version}</version>
<configuration>
<goalPrefix>screenshot</goalPrefix>
<sourceCodeURL>${project.scm.url}/src/main/java</sourceCodeURL>
<javaDocImageScale>0.6</javaDocImageScale>
<locales>
<localeSpec> (1)
<language>en</language>
<country>US</country>
</localeSpec>
</locales>
</configuration>
<executions>
<execution>
<id>create-gallery</id>
<phase>verify</phase> (2)
<goals>
<goal>gallery</goal>
</goals>
<configuration>
<outputDirectory>${screenshotGalleryTarget}</outputDirectory> (3)
</configuration>
</execution>
</executions>
</plugin>
.
.
.
</plugins>
</pluginManagement>
1 | In a previous version of the gallery goal it was possible to specify multiple locales and
get a side-by-side view of screenshots. This feature is unavailable for now. Pick one locale
at the time. |
2 | In this example the screenshot gallery is created in the default maven build cycle. The modules that
provides gallery content are build before the gh-pages module by adding them as dependencies to the gh-pages module.
You can exclude the gallery generation from the build cycle by specify <phase>none</phase> and then execute with the
command:
|
3 | Specify a path to the directory where the gallery goal aggregates screenshots from different modules.
Screenshot images are stored in a separate directory for each module. |
Add a dependency element to the dependencyManagement part
<dependencyManagement>
<dependencies>
<dependency>
<groupId>se.bluebrim.maven.plugin</groupId>
<artifactId>screenshot-maven-plugin-api</artifactId>
<version>${screenshot-maven-plugin.version}</version>
<scope>test</scope>
</dependency>
.
.
.
</dependencies>
</dependencyManagement>
2. Configuration in module pom
Add the following to the pom
files of modules containing Swing classes that you like to generate screenshots of.
<dependencies>
<dependency>
<groupId>se.bluebrim.maven.plugin</groupId>
<artifactId>screenshot-maven-plugin-api</artifactId>
</dependency>
.
.
.
</dependencies>
The configuration above makes it possible to annotate test class method with @Screenshot
.
Since its most convenient to run the javadoc goal from command line you don’t have to
add any plugin configuration in the build section. To run the gallery
as part of your Maven build
add the following in the build section:
<plugins>
<plugin>
<groupId>se.bluebrim.maven.plugin</groupId>
<artifactId>screenshot-maven-plugin</artifactId>
</plugin>
</plugins>
3. Add a gh-pages module
To create an aggregated screenshot gallery you need a common output directory for all modules that
provides content to the gallery. Create a separate module and use its target
directory for this purpose.
That module also contains the AsciiDoc configuration necessary to
process the generated Asciidoc to html. The gh-pages
module in this project combines generated and
handwritten AsciiDoc and publish it on GitHub pages.
Since other modules writes to the target
directory of the gh-pages
module before the gh-pages
module
is build, we must skip the cleaning by adding the following in the pom
file:
<build>
<plugins>
<plugin>
<artifactId>maven-clean-plugin</artifactId>
<configuration>
<skip>true</skip>
</configuration>
</plugin>
.
.
</plugins>
</build>
Instead the cleaning of the gh-pages
module is done at the beginning of the build by adding the following to the
root parent pom
file:
<build>
<plugins>
<plugin>
<artifactId>maven-clean-plugin</artifactId>
<configuration>
<filesets>
<fileset>
<directory>gh-pages/target</directory>
<includes>
<include>**/*</include>
</includes>
</fileset>
</filesets>
</configuration>
</plugin>
.
.
</plugins>
</build>
4. Test your configuration
Verify the configuration by adding the following classes to one of those modules:
In src/main/java
add:
package se.bluebrim.maven.plugin.screenshot.example;
import java.awt.Color;
import javax.swing.BorderFactory;
import javax.swing.ImageIcon;
import javax.swing.JLabel;
import javax.swing.JPanel;
/**
* The classic "Hello World" used to demonstrate the Screenshot Maven Plugin.
* The camera image was found at: http://wefunction.com/2008/07/function-free-icon-set
* <br>
* <img src="doc-files/HelloWorldPanel.png" alt="Screenshot image of class: HelloWorldPanel">
*
* @author Goran Stack
*
*/
@SuppressWarnings("serial")
public class HelloWorldPanel extends JPanel {
public HelloWorldPanel()
{
JLabel label = new JLabel("Hello World from Screenshot Maven Plugin");
ImageIcon icon;
icon = new ImageIcon(getClass().getResource("camera.png"));
label.setIcon(icon);
label.setFont(label.getFont().deriveFont(32f));
label.setForeground(Color.DARK_GRAY);
add(label);
setBorder(BorderFactory.createCompoundBorder(BorderFactory.createLineBorder(Color.RED.darker(), 4), BorderFactory.createEmptyBorder(60, 20, 60, 20)));
}
}
In src/test/java add:
package se.bluebrim.maven.plugin.screenshot.example;
import javax.swing.JComponent;
import se.bluebrim.maven.plugin.screenshot.Screenshot;
public class HelloWorldPanelTest {
@Screenshot
public JComponent createScreenShot()
{
return new HelloWorldPanel();
}
}
If you are using Eclipse adding these classes are very simple. Just copy the source from this page and paste it into the
src/main
or src/test
folder in the Package Explorer view. Eclipse will create the package and the class for you.
From the command line run:
mvn screenshot:javadoc
A doc-files folder containing a HelloWorldPanel.png
is created at the same location as the source code for
HelloWorldPanel class.
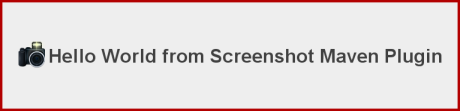
The screenshot plugin detect the missing img tag in the Javadoc of HelloWorldPanel
class and writes to the console.
[INFO] Missing "<img src="doc-files/HelloWorldPanel.png">" in class:se.bluebrim.maven.plugin.screenshot.example.HelloWorldPanel
To include the screenshot in the Javadoc of the HelloWorldPanel class copy the image tag from Maven console and paste into the Javadoc. You should now be able to see the image as part of the Javadoc.
5. Deploy content to GitHub pages
Travis has build in support for GitHub pages deployment. If you use a CI without this feature its also possible to use a script like this:
#!/bin/bash
cd gh-pages/target/generated-docs (1)
git init
git remote add origin git@github.com:goranstack/screenshot-maven-plugin.git (2)
git add .
git commit -m "Updated generated doc"
git push --force origin master:gh-pages (3)
rm -rf .git
1 | Specify a module with a build output to be published on GitHub pages |
2 | Specify your GitHub repo |
3 | Use force push to completely replace old content with new |